Getting started with pytest Course
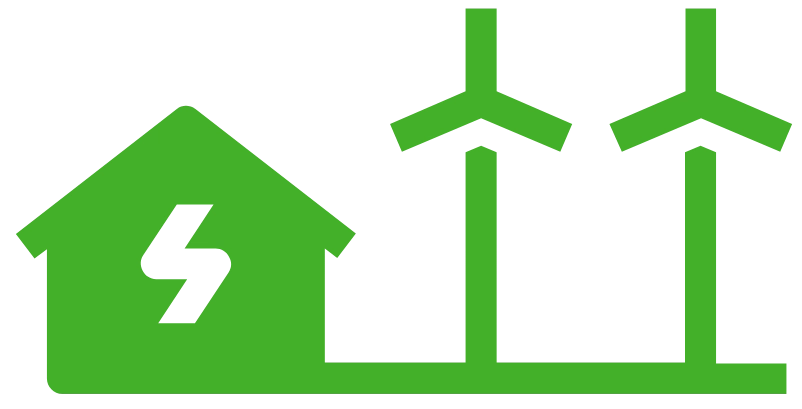
Course Summary
In Getting started with pytest, you'll do so much more than just get started. You'll learn the five super powers of pytest: simple test functions, fixtures, parametrization, markers, and plugins. With this knowledge, you'll be able to test more effectively and efficiently, whenever you need to write software tests.
What students are saying
Source code and course GitHub repository
github.com/talkpython/getting-started-with-pytest-courseWhat will you learn?
In this course, you will:
- Build clean, readable, well structured test functions.
- Utilize fixtures to hold setup, teardown, and test data.
- Share fixtures between tests and between test files.
- Trace test execution through tests and fixtures to help debug tests.
- Use test parametrization to create reams of test cases with a small number of test functions.
- Identify test parametrizations cleanly with test ids.
- Learn to skip tests with markers.
- Run subsets of tests using keywords, markers, test classes, and combinations of these techniques.
- Expand pytest functionality with pytest plugins.
- And lots more
- View the full course outline.
Who is this course for?
This course is for anyone who needs to test Python code, or anything reachable via Python code. Yep, pytest is being used to test APIs, web services, embedded systems, hardware, and of course Python packages, applications, and utilities.
Since many people are writing test code alongside their other work, the techniques presented in this course are focused on those that help you be efficient and effective with pytest.
Of course you can use these techniques if you are spending most of your time writing tests.
This course will be useful for software engineers of all flavors, including test engineers.
As for prerequisites, we assume:
- *No software testing experience required
- Familiarity with Python would be helpful.
Who am I? Why should you take my course?
My name is Brian Okken. There are a couple of reasons I'm especially qualified to teach you pytest.
- I wrote the first book on pytest, now in it's second edition, Python Testing with pytest.
- I host the #1 podcast on Python Testing, Test & Code.
- I'm experienced with pytest and Python testing. I've been using pytest to test Python applications and embedded C++ systems for over 10 years.
- I'm an experienced software engineer and lead engineer with 20+ years of industry experience. This is where I've learned to lean on efficient testing practices to help me write better applications faster.
Concepts backed by working code
Each chapter explores topics with working code. The code is all available to download or clone through GitHub. Each chapter is separated into directories.
While learning a topic, it's really important to run the code yourself, and explore it. Change the code, see what happens. Add new tests, break existing tests, have fun.
Follow along with subtitles and transcripts
Each course comes with subtitles and full transcripts. The transcripts are available as a separate searchable page for each lecture. They also are available in course-wide search results to help you find just the right lecture.
This course is delivered in very high resolution
This course is delivered in 1440p (4x the pixels as 720p). When you're watching the videos for this course, it will feel like you're sitting next to the instructor looking at their screen.
Every little detail, menu item, and icon is clear and crisp. Watch the introductory video at the top of this page to see an example.
Is this course based on Python 3 or Python 2?
This course is based upon Python 3. Python 2 is officially unsupported as of January 1st, 2020 and we believe that it would be ill-advised to teach or learn Python 2. This course is, and has always been, built around Python 3.
The time to act is now
pytest has become the defacto way we test Python software. If you are not up to speed with pytest and clean test practices, you'll be at a disadvantage. This course is focused on using the best techniques to provide a deep and meaningful understanding of software testing with pytest. Take the course, level up your productivity today.